Geofencing in Android
A Powerful Location-Based Technology for Your App Marketing Strategy
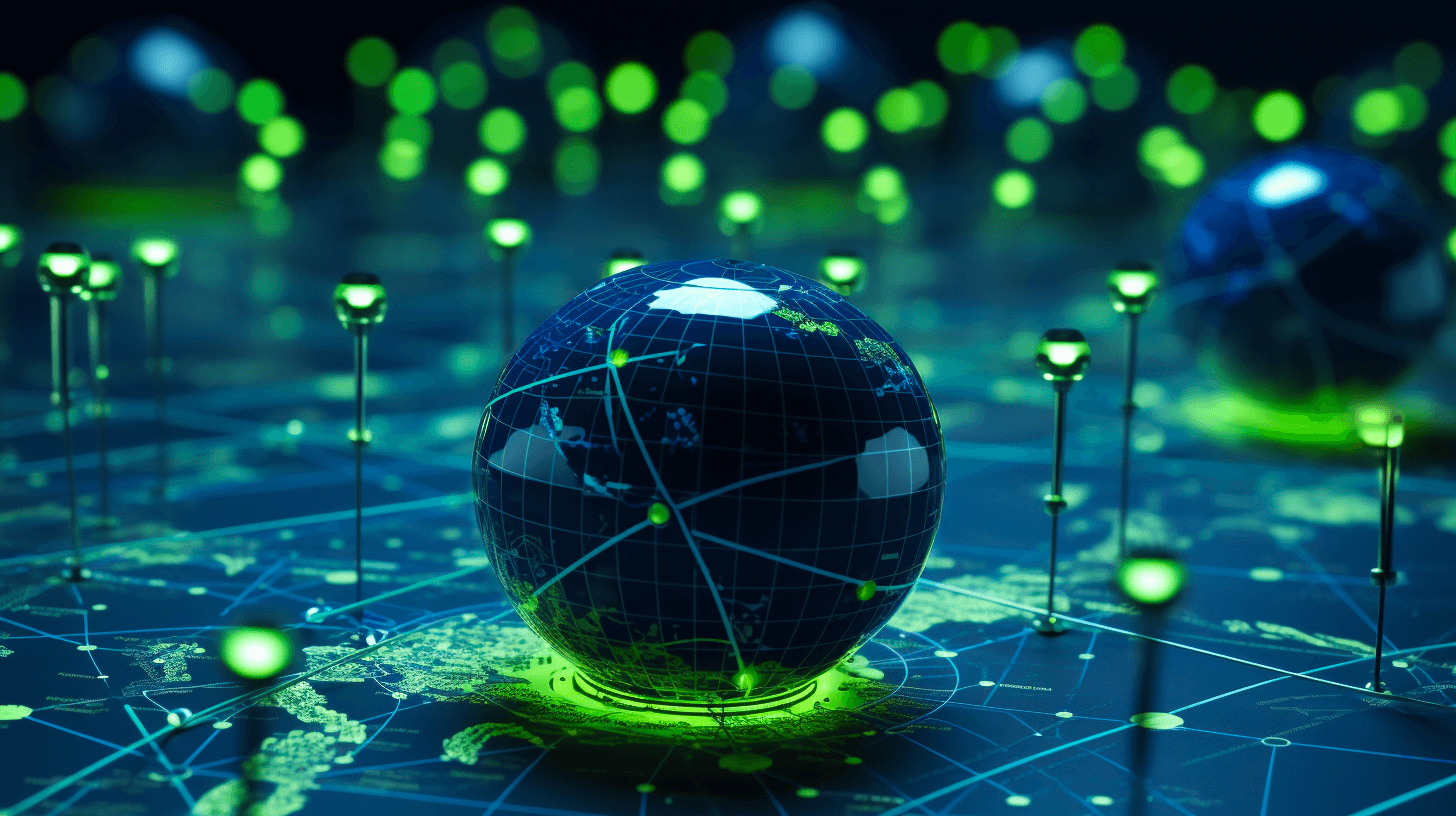
Imagine a world where your smartphone can automatically trigger actions and alerts based on your physical location. Geofencing is the technology that makes this scenario a reality. By drawing virtual boundaries around physical areas, businesses and app developers can provide users with targeted and context-aware content, transforming the way we interact with our devices.
What is Geofencing
Geofencing in mobile apps refers to the use of GPS, Wi-Fi, or Mobile 3G/4G data to send special messages, serve targeted advertisements, unlock offers, and/or send alerts to a user’s mobile phone when they go into or out of a specific geotagged area. The mobile app user gets a notification when they are close to a certain place or leave it.
In practical terms, geofencing is a virtual fence that enables apps to share or request information and perform certain actions based on data like GPS location and time.
You can track a user’s location through GPS, Bluetooth, and beacons, and there are three ways to utilize this technology for targeting users:
- Geotargeting - geotargeting is focused on delivering targeted advertising to users based on their location and interest or intent.
- Beaconing - beaconing is focused on transmitting targeted messages and information to nearby mobile devices.
- Geofencing - geofencing is focused on the virtual perimeter you build around a specific geographic location to deliver targeted messaging or enable app features.
Geofencing in Android
To implement Geofencing in Android apps, you need to follow below steps:
Step 1:
To use geofencing, your app must request the following permissions:
- ACCESS_FINE_LOCATION
- ACCESS_BACKGROUND_LOCATION (Android 10 (API level 29) or higher )
Step 2:
Add receiver in application tag of Manifest file.
<application
android:allowBackup="true">
<receiver android:name=".GeofenceBroadcastReceiver"/>
<application/>
Step 3:
Create a list of geofences to be tracked.
geofenceList.add(Geofence.Builder()
.setRequestId(entry.key)
// Set the circular region of this geofence.
.setCircularRegion(
entry.value.latitude,
entry.value.longitude,
Constants.GEOFENCE_RADIUS_IN_METERS
)
// Set the expiration duration of the geofence. This geofence gets automatically
// removed after this period of time.
.setExpirationDuration(Constants.GEOFENCE_EXPIRATION_IN_MILLISECONDS)
// Set the transition types of interest. Alerts are only generated for these
// transition. We track entry and exit transitions in this sample.
.setTransitionTypes(Geofence.GEOFENCE_TRANSITION_ENTER or Geofence.GEOFENCE_TRANSITION_EXIT)
// Create the geofence.
.build())
Step 4:
Create geofence request and pending intent of geofence transition.
class MainActivity : AppCompatActivity() {
private val geofencePendingIntent: PendingIntent by lazy {
val intent = Intent(this, GeofenceBroadcastReceiver::class.java)
// We use FLAG_UPDATE_CURRENT so that we get the same pending intent back when calling
// addGeofences() and removeGeofences().
PendingIntent.getBroadcast(this, 0, intent, PendingIntent.FLAG_UPDATE_CURRENT)
}
private fun getGeofencingRequest(): GeofencingRequest {
return GeofencingRequest.Builder().apply {
setInitialTrigger(GeofencingRequest.INITIAL_TRIGGER_ENTER)
addGeofences(geofenceList)
}.build()
}
}
Step 5:
Add geofences on GeofencingClient with success and failure listeners.
geofencingClient?.addGeofences(getGeofencingRequest(), geofencePendingIntent)?.run {
addOnSuccessListener {
// Geofences added
// ...
}
addOnFailureListener {
// Failed to add geofences
// ...
}
}
Step 6:
Now handle the geofence transition in your receiver.
class GeofenceBroadcastReceiver : BroadcastReceiver() {
// ...
override fun onReceive(context: Context?, intent: Intent?) {
val geofencingEvent = GeofencingEvent.fromIntent(intent)
if (geofencingEvent.hasError()) {
val errorMessage = GeofenceStatusCodes
.getStatusCodeString(geofencingEvent.errorCode)
Log.e(TAG, errorMessage)
return
}
// Get the transition type.
val geofenceTransition = geofencingEvent.geofenceTransition
// Test that the reported transition was of interest.
if (geofenceTransition == Geofence.GEOFENCE_TRANSITION_ENTER |
geofenceTransition == Geofence.GEOFENCE_TRANSITION_EXIT) {
// Get the geofences that were triggered. A single event can trigger
// multiple geofences.
val triggeringGeofences = geofencingEvent.triggeringGeofences
// Get the transition details as a String.
val geofenceTransitionDetails = getGeofenceTransitionDetails(
this,
geofenceTransition,
triggeringGeofences
)
// Send notification and log the transition details.
sendNotification(geofenceTransitionDetails)
Log.i(TAG, geofenceTransitionDetails)
} else {
// Log the error.
Log.e(TAG, getString(R.string.geofence_transition_invalid_type,
geofenceTransition))
}
}
}
Done, you have successfully added geofences and listened to the transitions.
Limitations:
You can have multiple active geofences, with a limit of 100 per app, per device user.
Best practices:
- Always remove geofences when the user logs out, or when not needed
- Opt for extended time intervals for Geofence enter/exit notifications to conserve device battery life. Ideally, these intervals should be set at a minimum of 5 minutes.
- Try to use a larger radius for geofences to reduce app checks for entrance or exit. Optimal radius size should be between 100 - 150 meters.
- Clearly explain to your users why your app uses geofencing
Benefits of Geofencing
There are multiple benefits of using and implementing Geofencing in Android apps, let’s discuss some of them.
Enhanced Targeted Marketing:
Geofencing allows businesses to send location-specific promotions and advertisements to users when they enter a defined geographic area, which can help increase conversions.
Improved Customer Engagement:
Geofencing enables personalized interactions with customers by delivering relevant content or offers based on their physical location, and can lead to improved customer loyalty.
Increased Traffic:
Retailers can attract more customers to their physical stores by sending real-time offers or promotions when potential customers are nearby.
Geographically Relevant Information:
Geofencing provides users with location-based information, such as local events, traffic updates, and nearby points of interest.
Asset and Fleet Management:
Businesses can track and manage the movement of vehicles and assets, improving efficiency and security.
Geolocation Analytics:
Businesses can gain valuable insights into user behaviour and preferences through geofencing data and analytics.
Personalized User Experiences:
Geofencing enables the delivery of personalized content, enhancing user experiences and satisfaction.
Geofencing impact on device Battery
Geofencing primarily relies on location services which can impact the device's battery life. There are factors to consider that can impact battery life:
Location Updates Frequency:
The more frequently your geofencing system requests location updates, the more it can drain the battery.
Accuracy Level:
Geofencing can use different methods for location detection, such as GPS, Wi-Fi, or cell towers. Using high-accuracy methods (e.g., GPS) consumes more power than low-accuracy methods (e.g., Wi-Fi).
Background Processing:
Geofencing often works in the background even when the app is not in the foreground, so it can impact battery performance.
Proximity to Geofence Boundaries:
Frequent transitions in and out of geofence boundaries can result in more location updates and potentially increased battery usage.
To minimize the impact on the device's battery life, follow these best practices:
- Use a proper location update strategy that balances accuracy and frequency.
- Be mindful of the geofence radius and trigger conditions.
- Periodically adjust the geofence radius based on user behavior and the application's requirements.
- Implement in-app logic to reduce unnecessary location updates when the app is not in active use.
- Take advantage of Android's location services features, like location batching and fused location provider, for efficient location updates.
In conclusion, geofencing is a powerful technology available to Android app developers that opens up a world of possibilities for location-based services. Whether you're creating a retail app that sends special offers to customers as they enter your store, or a safety app that notifies users when they enter or leave specific areas, geofencing provides the tools you need to enhance user experiences and create innovative solutions. By mastering the principles of geofencing and understanding its potential, you can take your Android app development to the next level and offer users a more personalized and context-aware experience.